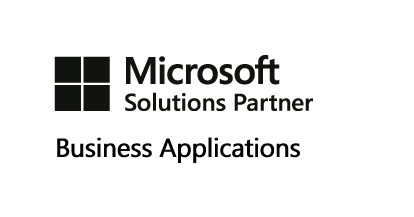
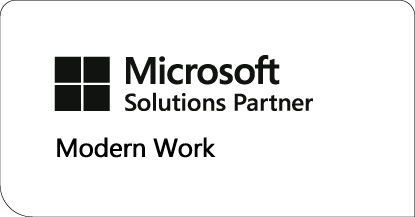

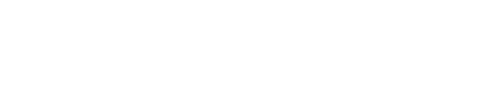
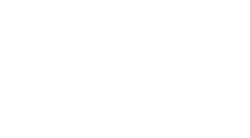
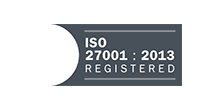
We recently had a request from a client to modify their business process flow and it seemed worthwhile to share our experience and the lessons learned in this scenario.
This business process flow was incredibly complicated and was designed to move the record between various staff members who each performed a separate task (with one stage per task). However, at any point within this process, a staff member could update the record and stop all further stages and they used a field on the business process flow to manage this. This had been working well for them, but they wanted to move the field from the business process flow to the header and still wanted to keep the functionality.
So, they had the same field on multiple stages of their business process flow where ALL instances needed to be hidden.
If you have a quick look on the internet, you’ll see lots of blog posts about hiding a field in a business process flow using JavaScript. So here it is again!
function hideFields(executionContext)
{
var formContext = executionContext.getFormContext();
formContext.getControl('header_process_tm_fieldname').setVisible(false);
}
If you call the ‘hideFields’ function from your form, then the field with the schema name ‘tm_fieldname’ will be hidden on the business process flow. As I say, I’m not breaking any new ground here. If you run this code, it hides the first one, so all the others remain. How do you do this? I couldn’t find anything out there that could help me.
After a bit of experimentation with trial and error, the answer, as it turns out, is quite simple – and it’s not just a case of repeating that last line multiple times (trust me, I tried that too!).
I updated the function to the one below:
function hideFields(executionContext)
{
var formContext = executionContext.getFormContext();
formContext.getControl('header_process_tm_fieldname').setVisible(false);
formContext.getControl('header_process_tm_fieldname_1').setVisible(false);
...
formContext.getControl('header_process_tm_fieldname_8').setVisible(false);
}
Yep, it’s just a case of appending ‘_1’, ‘_2’, etc to the field name and it will pick that instance of the field. I was delighted when I tested this and it worked but, when I opened a closed record, it started to give me a script error.
It turns out that it’s a simple case of referring to fields that are no longer there. As the business process branches off, the fields are no longer available, so the code is trying to update the visibility of fields which aren’t available which is never a good thing. It’s then just a case of adding an ‘if’ around each of the ‘setVisible’ statements to check if the field is actually there:
function hideFields(executionContext)
{
var formContext = executionContext.getFormContext();
if (formContext.getControl('header_process_tm_fieldname') != null)
formContext.getControl('header_process_tm_fieldname').setVisible(false);
if (formContext.getControl('header_process_tm_fieldname_1') != null)
formContext.getControl('header_process_tm_fieldname_1').setVisible(false);
...
if (formContext.getControl('header_process_tm_fieldname_8') != null)
formContext.getControl('header_process_tm_fieldname_8').setVisible(false);
}
That’s it! This isn’t something that’s cropped up a lot over the years but, when I did need help, I found absolutely nothing out there to help me. Hopefully, this will help you.